Creating a QR Menu Generator Application in Next.js with TypeScript
Prerequisites
Before we begin, make sure you have the following installed on your machine:
Node.js (v14 or higher)
npm or yarn
A code editor (we recommend Visual Studio Code)
Step 1: Setting Up the Project
First, let's create a new Next.js project. Open your terminal and run the following command:
npx create-next-app@latest qr-menu-generator --typescript
Navigate to the project directory:
cd qr-menu-generator
Step 2: Installing Dependencies
We need to install a few dependencies for our project. Run the following command to install them:
npm install qrcode
The qrcode
package will help us generate QR codes.
Folder Structure
Here's the folder structure of the project:
scan-menu/ ├── src/ │ ├── app/ │ │ ├── generate/ │ │ │ └── page.tsx // The QR code generator page │ │ ├── api/ │ │ │ └── save-menu/ │ │ │ └── route.ts // The API route │ │ └── menu/ │ │ └── [id]/ │ │ └── page.tsx // Your dynamic menu page ├── public/ │ └── menus/ // Contains JSON files └── package.json
Step 3: Creating the API Route
Next, let's create an API route to save the menu data. Create a new file at app/api/save-menu/routes.ts
and add the necessary code to handle saving the menu data to a JSON file in the public/menus
directory.
import { NextResponse } from 'next/server'; import fs from 'fs'; import path from 'path'; export async function POST(request: Request) { try { const { restaurantName, dishes } = await request.json(); if (!restaurantName || !dishes) { return NextResponse.json( { success: false, message: 'Invalid data' }, { status: 400 } ); } // Generate a restaurant id (e.g., "gouri-tandoori-dhaba") const restaurantId = restaurantName .toLowerCase() .trim() .replace(/\s+/g, '-'); const menusDir = path.join(process.cwd(), 'public', 'menus'); // Ensure the menus directory exists if (!fs.existsSync(menusDir)) { fs.mkdirSync(menusDir, { recursive: true }); } const filePath = path.join(menusDir, `${restaurantId}.json`); // Write (or overwrite) the file so that duplicate files are not created fs.writeFileSync(filePath, JSON.stringify(dishes, null, 2)); return NextResponse.json({ success: true, id: restaurantId }); } catch (error) { console.error('Error saving menu:', error); return NextResponse.json( { success: false, message: 'Error saving menu' }, { status: 500 } ); } }
This API route handles saving the menu data to a JSON file in the public/menus
directory. It performs the following steps:
Parses the request body to extract the restaurant name and dishes.
Validates the input data.
Generates a unique restaurant ID by converting the restaurant name to lowercase and replacing spaces with hyphens.
Ensures the public/menus
directory exists, creating it if necessary.
Writes the menu data to a JSON file named after the restaurant ID.
Returns a success response with the restaurant ID.
Step 4: Creating the Generate QR Page
Now, let's create the main page for generating QR codes. Create a new file at app/generate/page.tsx
and add the necessary code to allow users to enter restaurant details and dishes, and generate a QR code for the menu.
'use client'; import { useState } from 'react'; import QRCode from 'qrcode'; interface Dish { name: string; price: string; category: string; type: 'Veg' | 'Non-Veg'; } const defaultDish: Dish = { name: '', price: '', category: 'Main Course', type: 'Veg', }; export default function GenerateQR() { const [restaurantName, setRestaurantName] = useState(''); const [currentDish, setCurrentDish] = useState<Dish>(defaultDish); const [dishes, setDishes] = useState<Dish[]>([]); const [qrCode, setQrCode] = useState(''); const [showQrModal, setShowQrModal] = useState(false); // Add the current dish to the dish list and reset the form const addDish = () => { if (!currentDish.name.trim() || !currentDish.price.trim()) { alert('Please fill in dish name and price.'); return; } setDishes([...dishes, currentDish]); setCurrentDish(defaultDish); }; // Remove a dish from the list const removeDish = (index: number) => { setDishes(dishes.filter((_, i) => i !== index)); }; // Generate the QR code after validating inputs and saving the menu const handleGenerateQR = async () => { if (!restaurantName.trim()) { alert('Please enter a restaurant name.'); return; } if (dishes.length === 0) { alert('Please add at least one dish.'); return; } try { const response = await fetch('/api/save-menu', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ restaurantName, dishes }), }); const data = await response.json(); if (data.success) { const qrData = `${window.location.origin}/menu/${data.id}`; const qrImage = await QRCode.toDataURL(qrData); setQrCode(qrImage); setShowQrModal(true); } else { alert('Error saving menu'); } } catch (error) { console.error('Error generating QR:', error); alert('Something went wrong.'); } }; const downloadQRCode = () => { const canvas = document.createElement('canvas'); const context = canvas.getContext('2d'); if (!context) return; const qrImg = new Image(); qrImg.src = qrCode; qrImg.onload = () => { // Set canvas size (adjust as needed) canvas.width = 300; canvas.height = 360; // Draw background context.fillStyle = '#ffffff'; context.fillRect(0, 0, canvas.width, canvas.height); // Add header text context.fillStyle = '#000000'; context.font = '20px Arial'; context.textAlign = 'center'; context.fillText('Your Menu QR Code', canvas.width / 2, 30); // Draw QR Code context.drawImage(qrImg, 25, 50, 250, 250); // Convert canvas to image and trigger download const link = document.createElement('a'); link.download = 'menu-qr-code.png'; link.href = canvas.toDataURL('image/png'); link.click(); }; }; return ( <div className="min-h-screen bg-gradient-to-r from-green-400 to-blue-500 flex flex-col items-center py-8 px-4"> <div className="bg-white rounded-lg shadow-xl max-w-3xl w-full p-8"> <h1 className="text-4xl font-bold text-center mb-6">Create Your Restaurant Menu</h1> {/* Restaurant Name Input */} <div className="mb-6"> <label className="block text-xl font-medium text-gray-700 mb-2"> Restaurant Name </label> <input type="text" placeholder="Enter restaurant name..." value={restaurantName} onChange={(e) => setRestaurantName(e.target.value)} className="w-full p-3 border border-gray-300 rounded-lg focus:outline-none focus:ring-2 focus:ring-blue-400" /> </div> {/* Dish Addition Form */} <div className="mb-6"> <h2 className="text-2xl font-semibold text-gray-800 mb-4">Add a Dish</h2> <div className="grid grid-cols-1 sm:grid-cols-2 gap-4 mb-4"> <div> <label className="block text-sm font-medium text-gray-600 mb-1">Dish Name</label> <input type="text" placeholder="e.g. Paneer Tikka" value={currentDish.name} onChange={(e) => setCurrentDish({ ...currentDish, name: e.target.value }) } className="w-full p-2 border border-gray-300 rounded-md focus:outline-none focus:ring-2 focus:ring-blue-300" /> </div> <div> <label className="block text-sm font-medium text-gray-600 mb-1">Price</label> <input type="text" placeholder="e.g. $10" value={currentDish.price} onChange={(e) => setCurrentDish({ ...currentDish, price: e.target.value }) } className="w-full p-2 border border-gray-300 rounded-md focus:outline-none focus:ring-2 focus:ring-blue-300" /> </div> </div> <div className="grid grid-cols-1 sm:grid-cols-2 gap-4 mb-4"> <div> <label className="block text-sm font-medium text-gray-600 mb-1">Category</label> <select value={currentDish.category} onChange={(e) => setCurrentDish({ ...currentDish, category: e.target.value }) } className="w-full p-2 border border-gray-300 rounded-md focus:outline-none focus:ring-2 focus:ring-blue-300" > <option value="Appetizer">Appetizer</option> <option value="Main Course">Main Course</option> <option value="Dessert">Dessert</option> <option value="Beverage">Beverage</option> </select> </div> <div> <label className="block text-sm font-medium text-gray-600 mb-1">Type</label> <select value={currentDish.type} onChange={(e) => setCurrentDish({ ...currentDish, type: e.target.value as 'Veg' | 'Non-Veg', }) } className="w-full p-2 border border-gray-300 rounded-md focus:outline-none focus:ring-2 focus:ring-blue-300" > <option value="Veg">Veg</option> <option value="Non-Veg">Non-Veg</option> </select> </div> </div> <div className="text-right"> <button onClick={addDish} className="bg-green-500 hover:bg-green-600 text-white font-medium py-2 px-4 rounded transition duration-200" > + Add Dish </button> </div> </div> {/* Dish Preview */} {dishes.length > 0 && ( <div className="mb-6"> <h2 className="text-2xl font-semibold text-gray-800 mb-4">Dish Preview</h2> <div className="grid grid-cols-1 sm:grid-cols-2 gap-4"> {dishes.map((dish, index) => ( <div key={index} className="bg-gray-100 rounded-lg shadow p-4 flex items-center justify-between" > <div> <h3 className="text-xl font-bold text-gray-700">{dish.name}</h3> <p className="text-gray-600">{dish.price}</p> <p className="text-sm text-gray-500"> {dish.category} |{' '} {dish.type === 'Veg' ? '🍃 Veg' : '🍖 Non-Veg'} </p> </div> <button onClick={() => removeDish(index)} className="text-red-500 hover:text-red-700 font-bold text-2xl" title="Remove Dish" > × </button> </div> ))} </div> </div> )} {/* Generate QR Button */} <div className="text-center"> <button onClick={handleGenerateQR} className="bg-blue-600 hover:bg-blue-700 text-white font-bold py-3 px-8 rounded-lg transition duration-200" > Generate QR Code </button> </div> </div> {/* QR Code Modal */} {showQrModal && ( <div className="fixed inset-0 z-50 flex items-center justify-center bg-black bg-opacity-50"> <div className="bg-white rounded-lg p-8 shadow-xl max-w-sm w-full text-center relative animate-fadeIn"> <button onClick={() => setShowQrModal(false)} className="absolute top-2 right-2 text-gray-600 hover:text-gray-800 font-bold text-2xl" title="Close" > × </button> <h2 className="text-2xl font-semibold mb-4">Your Menu QR Code</h2> <img src={qrCode} alt="QR Code" className="mx-auto border p-4 rounded" /> <button onClick={downloadQRCode} className="bg-green-500 hover:bg-green-600 text-white font-medium py-2 px-4 rounded mt-4" > Download QR Code </button> </div> </div> )} </div> ); }
This page allows users to enter restaurant details and dishes, and generates a QR code for the menu. It includes the following features:
Form inputs for entering the restaurant name and dish details.
Functions to add and remove dishes from the list.
A function to handle generating the QR code, which involves:
Sending the menu data to the API route.
Generating a QR code for the menu URL.
Displaying the QR code in a modal.
A function to download the generated QR code as an image.
Step 5: Creating the Menu Display Page
Finally, let's create a page to display the menu based on the restaurant ID. Create a new file at app/menu/[id]/page.tsx
and add the necessary code to read the menu data from the JSON file and display it.
This page displays the menu based on the restaurant ID. It performs the following steps:
Reads the menu data from the corresponding JSON file in the public/menu
s directory.
Displays the menu items in a styled layout.
import fs from 'fs'; import path from 'path'; interface MenuItem { name: string; price: string; category: string; type: 'Veg' | 'Non-Veg'; } interface Props { params: { id: string }; } // Pre-generate static params for each menu file export async function generateStaticParams() { const menusDir = path.join(process.cwd(), 'public', 'menus'); const files = fs.readdirSync(menusDir); return files.map((file) => ({ id: file.replace('.json', ''), })); } // Helper function to convert strings to Title Case function toTitleCase(str: string): string { return str.replace(/\w\S*/g, (txt) => txt.charAt(0).toUpperCase() + txt.substr(1).toLowerCase() ); } export default async function MenuPage({ params }: Props) { const { id } = params; const menusDir = path.join(process.cwd(), 'public', 'menus'); const filePath = path.join(menusDir, `${id}.json`); // If the JSON file doesn't exist, show a friendly message. if (!fs.existsSync(filePath)) { return ( <div className="container mx-auto p-8 text-center text-2xl text-gray-800 dark:text-gray-100"> Menu not found! </div> ); } const menuData: MenuItem[] = JSON.parse(fs.readFileSync(filePath, 'utf-8')); // Group menu items by category. const groupedByCategory = menuData.reduce((acc: Record<string, MenuItem[]>, item) => { const key = item.category; if (!acc[key]) { acc[key] = []; } acc[key].push(item); return acc; }, {}); return ( <div className="min-h-screen bg-gradient-to-br from-teal-300 to-blue-300 dark:from-gray-900 dark:to-gray-800 py-10 px-4"> <div className="max-w-4xl mx-auto bg-white dark:bg-gray-800 rounded-xl shadow-2xl p-8"> {/* Restaurant Name in Title Case */} <h1 className="text-4xl font-extrabold text-center mb-10 text-gray-800 dark:text-gray-100"> {toTitleCase(id.replace(/-/g, ' '))}'s Menu </h1> {/* Render each category */} {Object.entries(groupedByCategory).map(([category, items]) => { // Treat "dessert" as a special case: all desserts are Veg by default. const isDessert = category.toLowerCase() === 'dessert'; if (isDessert) { return ( <div key={category} className="mb-10"> <h2 className="text-3xl font-bold border-b-2 border-gray-400 pb-2 mb-6 text-gray-800 dark:text-gray-100"> {toTitleCase(category)} </h2> <div className="grid grid-cols-1 sm:grid-cols-2 gap-6"> {items.map((item, index) => ( <div key={index} className="bg-white dark:bg-gray-700 border border-gray-200 dark:border-gray-600 rounded-xl p-6 transition transform hover:-translate-y-1 hover:shadow-2xl duration-300" > <h3 className="text-2xl font-bold text-gray-800 dark:text-gray-100"> {item.name} </h3> <p className="text-lg text-gray-600 dark:text-gray-300 mt-2">₹{item.price}</p> </div> ))} </div> </div> ); } else { // For other categories, split items into Veg and Non-Veg sections. const vegItems = items.filter((item) => item.type === 'Veg'); const nonVegItems = items.filter((item) => item.type === 'Non-Veg'); return ( <div key={category} className="mb-10"> <h2 className="text-3xl font-bold border-b-2 border-gray-400 pb-2 mb-6 text-gray-800 dark:text-gray-100"> {toTitleCase(category)} </h2> {vegItems.length > 0 && ( <div className="mb-8"> <h3 className="text-2xl font-semibold text-green-600 dark:text-green-400 mb-4 flex items-center"> <span className="mr-2">🍃</span> Veg </h3> <div className="grid grid-cols-1 sm:grid-cols-2 gap-6"> {vegItems.map((item, index) => ( <div key={index} className="bg-white dark:bg-gray-700 border border-gray-200 dark:border-gray-600 rounded-xl p-6 transition transform hover:-translate-y-1 hover:shadow-2xl duration-300" > <h3 className="text-2xl font-bold text-gray-800 dark:text-gray-100"> {item.name} </h3> <p className="text-lg text-gray-600 dark:text-gray-300 mt-2">₹{item.price}</p> </div> ))} </div> </div> )} {nonVegItems.length > 0 && ( <div> <h3 className="text-2xl font-semibold text-red-600 dark:text-red-400 mb-4 flex items-center"> <span className="mr-2">🍖</span> Non-Veg </h3> <div className="grid grid-cols-1 sm:grid-cols-2 gap-6"> {nonVegItems.map((item, index) => ( <div key={index} className="bg-white dark:bg-gray-700 border border-gray-200 dark:border-gray-600 rounded-xl p-6 transition transform hover:-translate-y-1 hover:shadow-2xl duration-300" > <h3 className="text-2xl font-bold text-gray-800 dark:text-gray-100"> {item.name} </h3> <p className="text-lg text-gray-600 dark:text-gray-300 mt-2">₹{item.price}</p> </div> ))} </div> </div> )} </div> ); } })} </div> </div> ); }
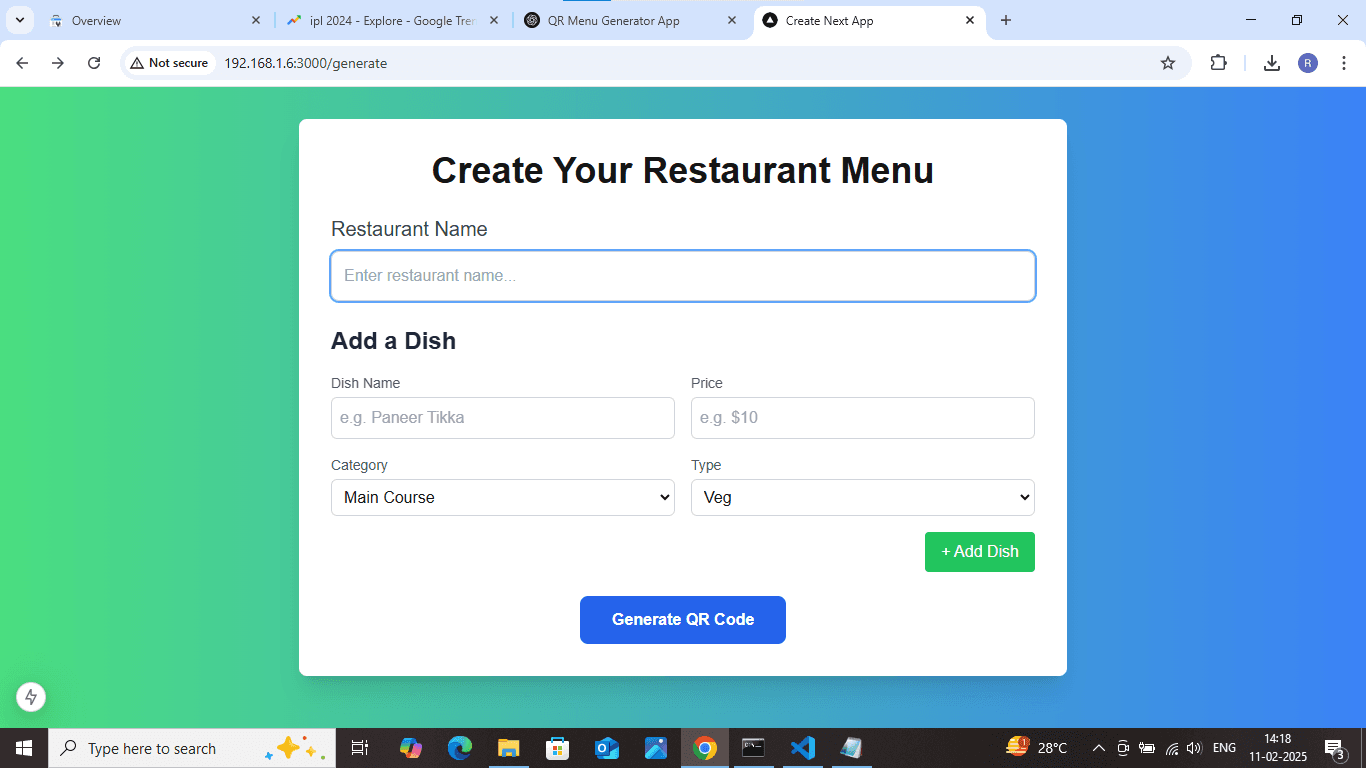
Create Menu Page
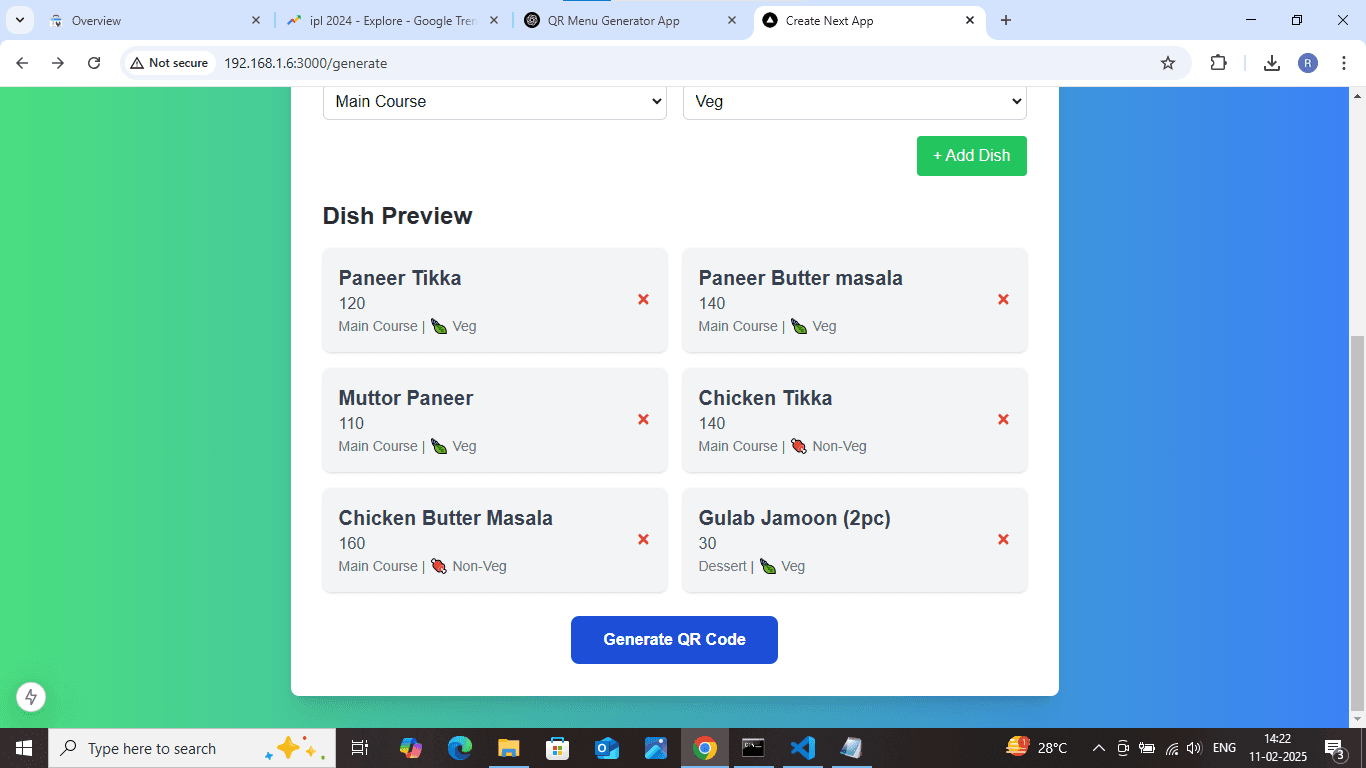
Dish Preview
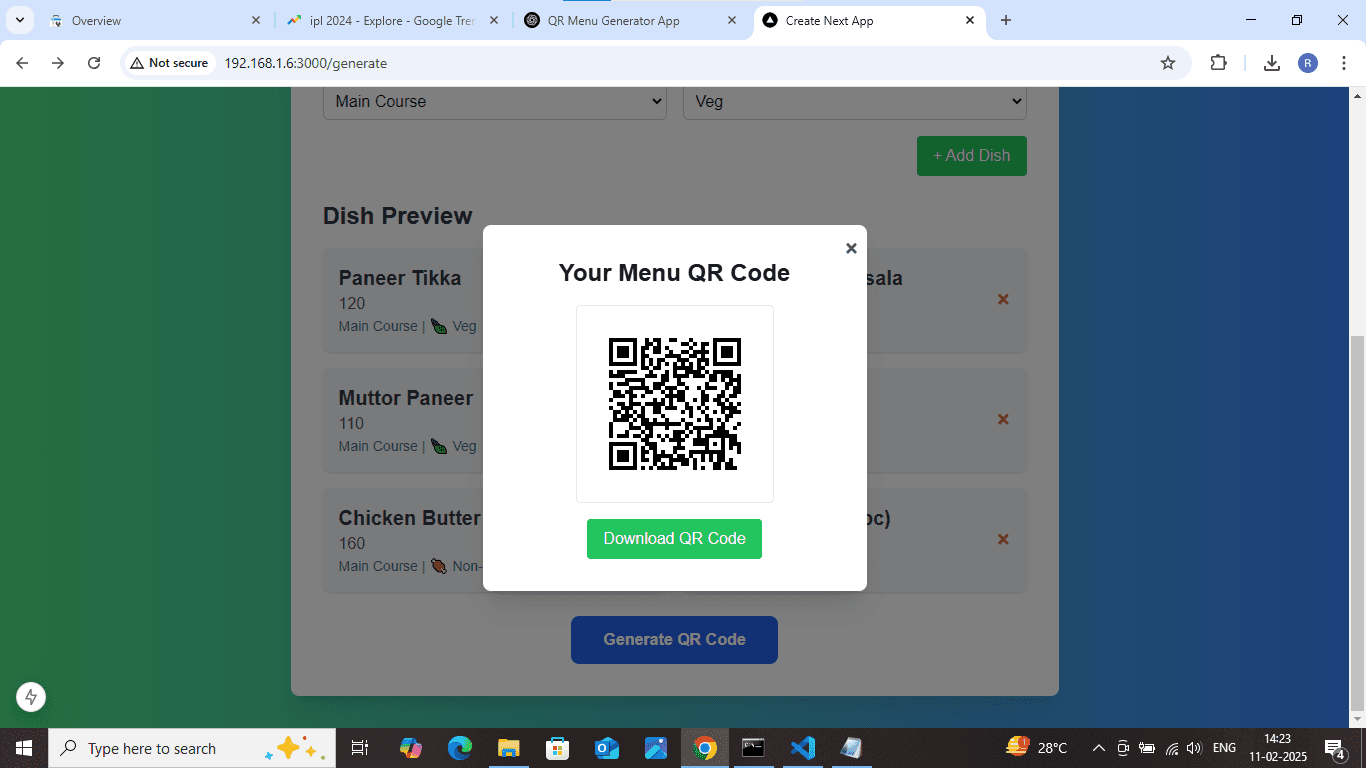
Generated QR
Example JSON File (public/menus/xyz-restaurant.json
)
This JSON file contains the menu data for a restaurant named "Maya Hotel and Restaurant". It includes details such as dish names, prices, categories, and types (Veg or Non-Veg).
[ { "name": "Paneer Tikka", "price": "120", "category": "Main Course", "type": "Veg" }, { "name": "Paneer Butter masala", "price": "140", "category": "Main Course", "type": "Veg" }, { "name": "Muttor Paneer", "price": "110", "category": "Main Course", "type": "Veg" }, { "name": "Chicken Tikka", "price": "140", "category": "Main Course", "type": "Non-Veg" }, { "name": "Chicken Butter Masala", "price": "160", "category": "Main Course", "type": "Non-Veg" }, { "name": "Gulab Jamoon (2pc)", "price": "30", "category": "Dessert", "type": "Veg" } ]
GitHub Repository
You can find the complete source code of this project on GitHub: https://github.com/raj-bhai/scan-menu.git
Conclusion
Congratulations! You have successfully created a QR menu generator application using Next.js and TypeScript. This application allows restaurant owners to create digital menus and generate QR codes for easy access by customers. If you are interested in purchasing this project with additional features, feel free to contact me.
Happy coding!